以下是一个使用 Python 和 Matplotlib 库绘制折线图的示例代码:
```python
#!/usr/bin/python
# -*- coding: utf-8 -*-
import matplotlib.pyplot as plt
import numpy as np
# 创建一个图形对象,设置图像尺寸、背景色和分辨率
fig = plt.figure(figsize=(10, 10), facecolor='blue', dpi=72)
# 添加一个子图
ax1 = fig.add_subplot(1, 1, 1)
# 设置图表标题(不支持中文)
ax1.set_title('Title')
# 设置坐标轴标签
ax1.set_xlabel('X Axis')
ax1.set_ylabel('Y Axis')
# 生成数据点
x = np.linspace(0, 2 * np.pi, 20)
y = np.sin(x)
y2 = np.cos(x)
# 绘制折线图
ax1.plot(x, y, label='SIN', color='y', linewidth=3, linestyle='--', marker='o')
ax1.plot(x, y2, label='COS', color='r')
# 添加图例
ax1.legend(loc='best')
# 添加注释
arrowprops = {'arrowstyle': '->', 'color': 'red'}
ax1.annotate('Max', xy=(np.pi / 2, 1), xytext=(np.pi / 2 + 0.5, 1), arrowprops=arrowprops)
# 显示图形
plt.show()
```
运行上述代码后,将生成一个包含正弦波和余弦波的折线图,效果如下所示:
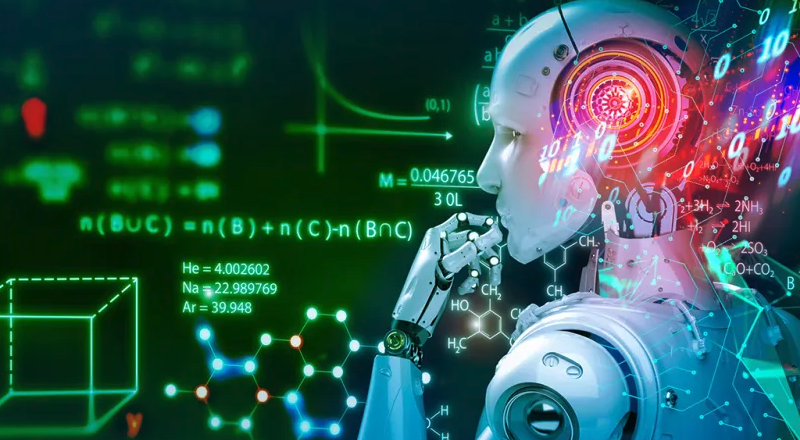
以上代码展示了如何使用 Matplotlib 库绘制折线图,并对图表的各种属性进行了详细的设置。更多关于 Python Matplotlib 的资料请参考相关文档和教程。