一、一般开发过程
Step1 : 建立数据库连接
以下是语法
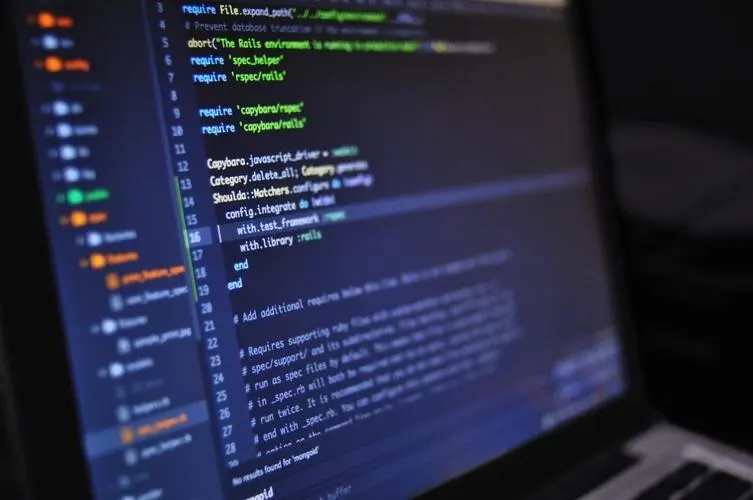
代码片段

Step2: 建立Statement对象
Statement基于数据库连接,稍后用来执行SQL查询 
Step3:执行SQL查询
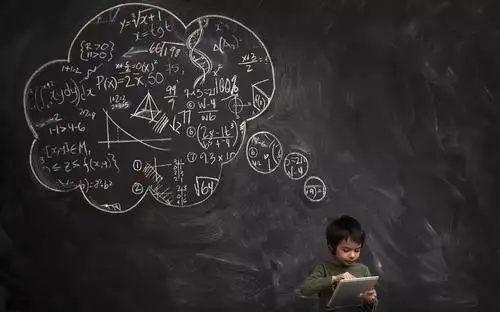
Step4: 处理结果集
方法ResultSet.next()的作用是向下移动一行,如果还有记录则返回true

读取数据
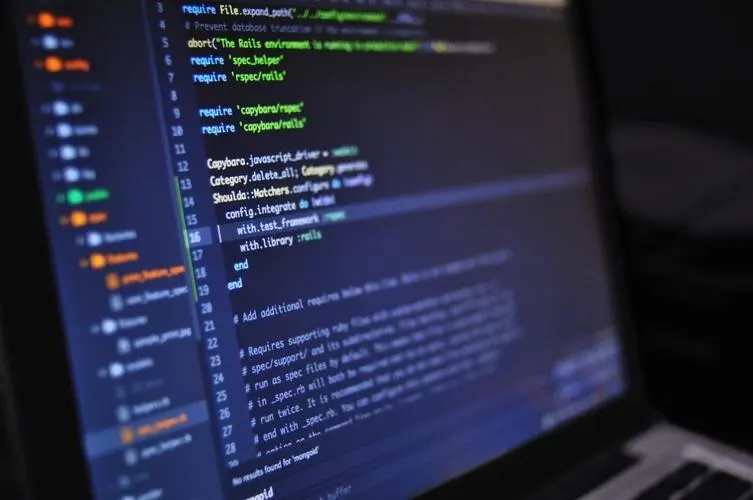
二、安装开发环境
Step1: 系统需求
create database if not exists demo;
use demo;
drop table if exists employees;
CREATE TABLE employees ( id int(11) NOT NULL AUTO_INCREMENT, last_name varchar(64) DEFAULT NULL, first_name varchar(64) DEFAULT NULL, email varchar(64) DEFAULT NULL, department varchar(64) DEFAULT NULL, salary DECIMAL(10,2) DEFAULT NULL, PRIMARY KEY (id) ) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1;
INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (1,'Doe','John','john.doe@foo.com', 'HR', 55000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (2,'Public','Mary','mary.public@foo.com', 'Engineering', 75000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (3,'Queue','Susan','susan.queue@foo.com', 'Legal', 130000.00);
INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (4,'Williams','David','david.williams@foo.com', 'HR', 120000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (5,'Johnson','Lisa','lisa.johnson@foo.com', 'Engineering', 50000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (6,'Smith','Paul','paul.smith@foo.com', 'Legal', 100000.00);
INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (7,'Adams','Carl','carl.adams@foo.com', 'HR', 50000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (8,'Brown','Bill','bill.brown@foo.com', 'Engineering', 50000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (9,'Thomas','Susan','susan.thomas@foo.com', 'Legal', 80000.00);
INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (10,'Davis','John','john.davis@foo.com', 'HR', 45000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (11,'Fowler','Mary','mary.fowler@foo.com', 'Engineering', 65000.00); INSERT INTO employees (id,last_name,first_name,email, department, salary) VALUES (12,'Waters','David','david.waters@foo.com', 'Legal', 90000.00);
Step2: 安装JDBC驱动
然后复制jdbc驱动到项目目录中
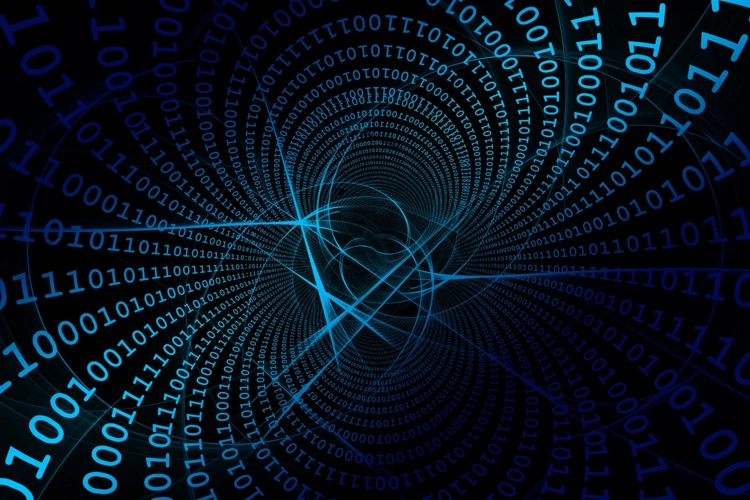
配置到类路径
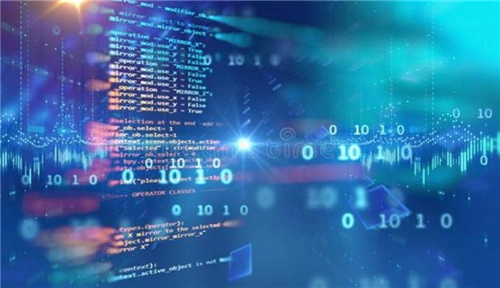
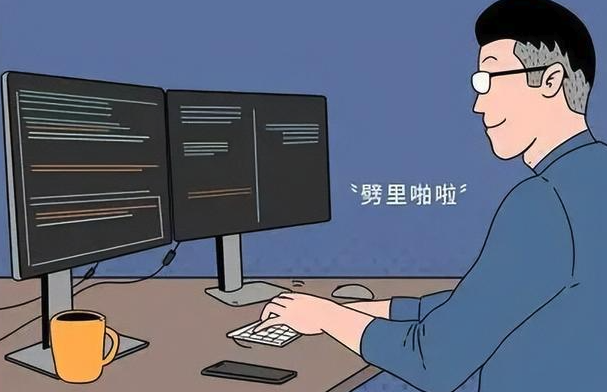
Step3 : 测试代码
import java.sql.*;
/**
*
* @author www.luv2code.com
*
*/
public class JdbcTest {
public static void main(String[] args) throws SQLException {
Connection myConn = null;
Statement myStmt = null;
ResultSet myRs = null;
try {
// 1\. Get a connection to database
myConn = DriverManager.getConnection("jdbc:mysql://192.168.1.8:3306/demo", "root" , "bihell.com");
System.out.println("Database connection successful!\n");
// 2\. Create a statement
myStmt = myConn.createStatement();
// 3\. Execute SQL query
myRs = myStmt.executeQuery("select * from employees");
// 4\. Process the result set
while (myRs.next()) {
System.out.println(myRs.getString("last_name") + ", " + myRs.getString("first_name"));
}
}
catch (Exception exc) {
exc.printStackTrace();
}
finally {
if (myRs != null) {
myRs.close();
}
if (myStmt != null) {
myStmt.close();
}
if (myConn != null) {
myConn.close();
}
}
}
}