在本示例中,我们将展示如何利用Java Swing框架构建一个简单的桌面应用程序,该程序能够以高可读性的形式动态显示当前系统的日期和时间。
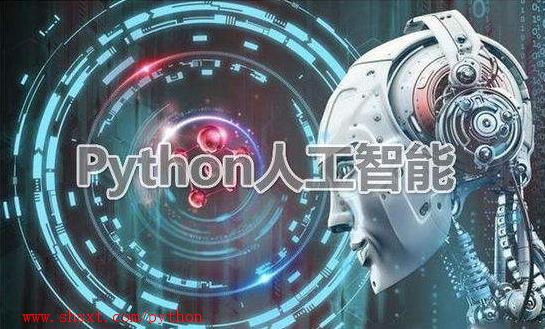
首先,我们需要导入必要的包:
import java.awt.Color;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.SimpleDateFormat;
import java.util.Date;
import javax.swing.JLabel;
import javax.swing.Timer;
import javax.swing.JFrame;
接下来是主类的定义:
public class RealTimeDisplay extends JFrame {
private static final long serialVersiOnUID= 1L;
public RealTimeDisplay() {
JLabel timeLabel = new JLabel();
timeLabel.setForeground(Color.BLUE);
timeLabel.setBounds(30, 0, 900, 130);
timeLabel.setFont(new Font("Microsoft YaHei", Font.BOLD, 80));
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(null);
this.setTitle("Real Time Clock");
this.setBounds(500, 200, 930, 200);
this.setVisible(true);
this.add(timeLabel);
initializeTimer(timeLabel);
}
private void initializeTimer(JLabel label) {
Timer timer = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
label.setText(sdf.format(new Date()));
}
});
timer.start();
}
public static void main(String[] args) {
new RealTimeDisplay();
}
}
上述代码创建了一个窗口,其中包含一个标签(JLabel
),用于显示格式化的当前日期和时间。通过设置一个每秒触发一次的Timer
,我们可以确保时间显示始终保持最新状态。
本示例不仅展示了如何使用Java Swing进行基本的GUI开发,还涵盖了如何处理时间格式化以及如何使用定时器来定期更新UI元素的内容。这对于初学者来说是一个很好的实践项目,有助于加深对Java GUI编程的理解。