【实例简介】一个很基础的Java实例,实现最基础的增删改查
【实例截图】
账号:01101 密码:1234
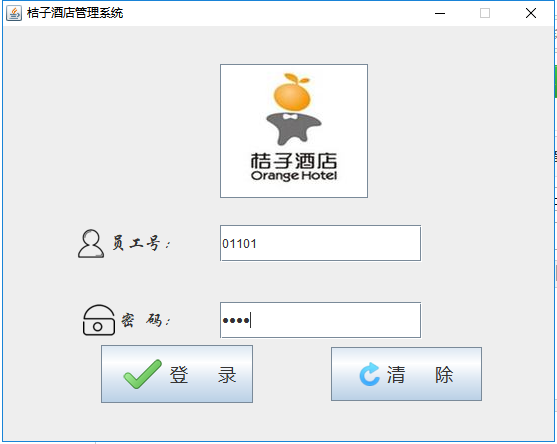
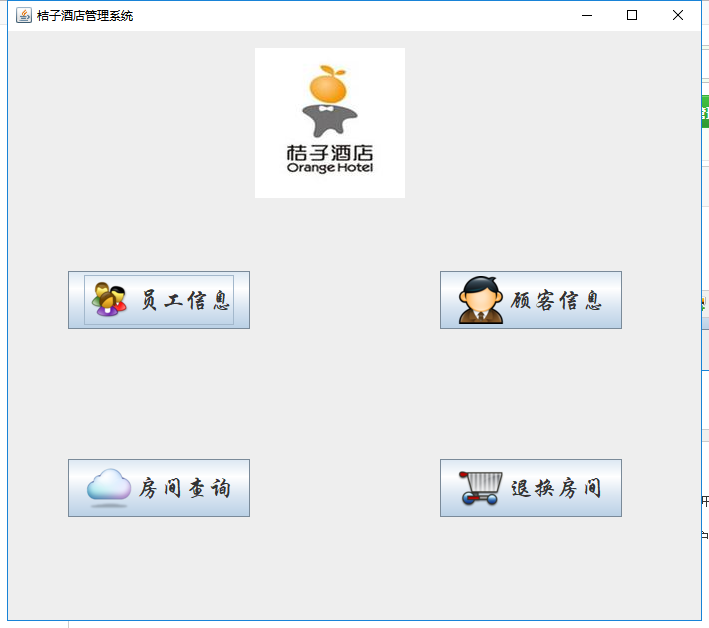
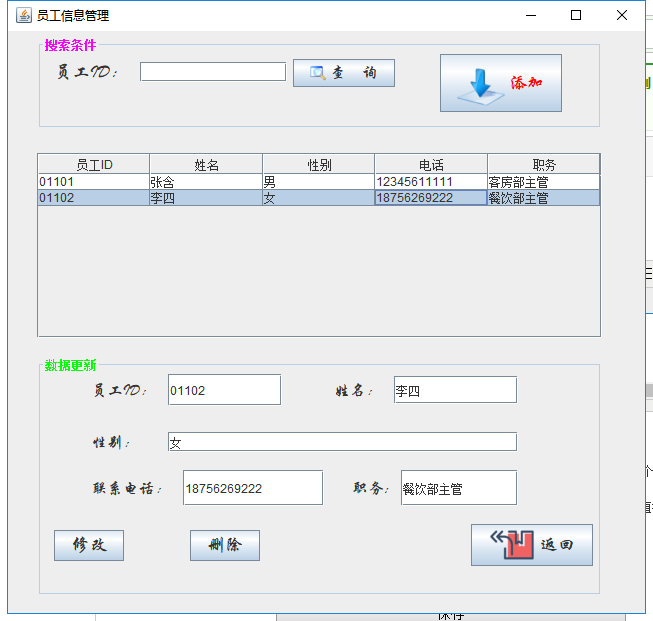
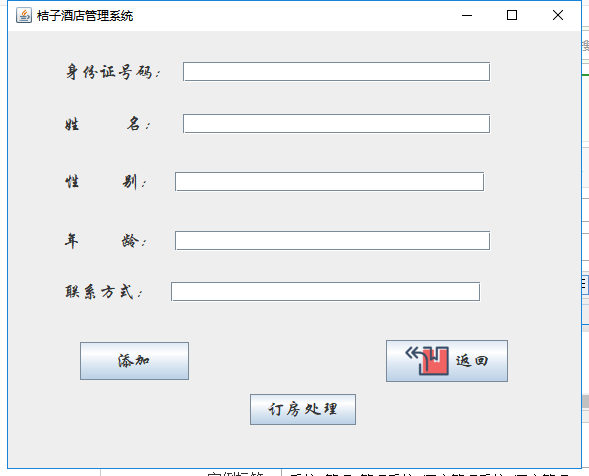
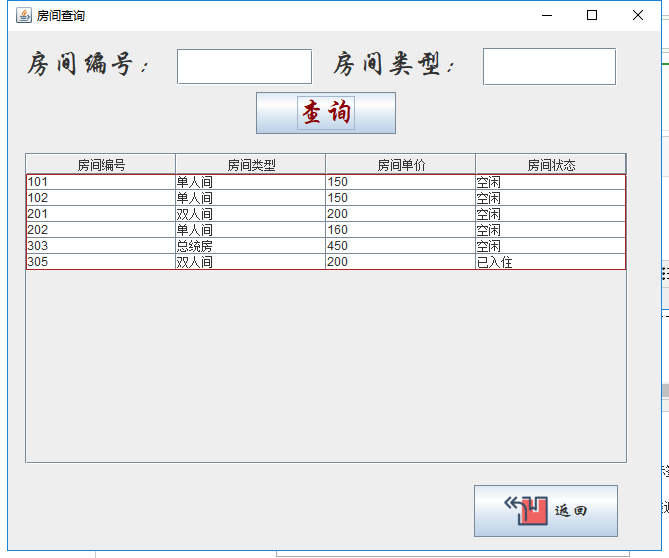
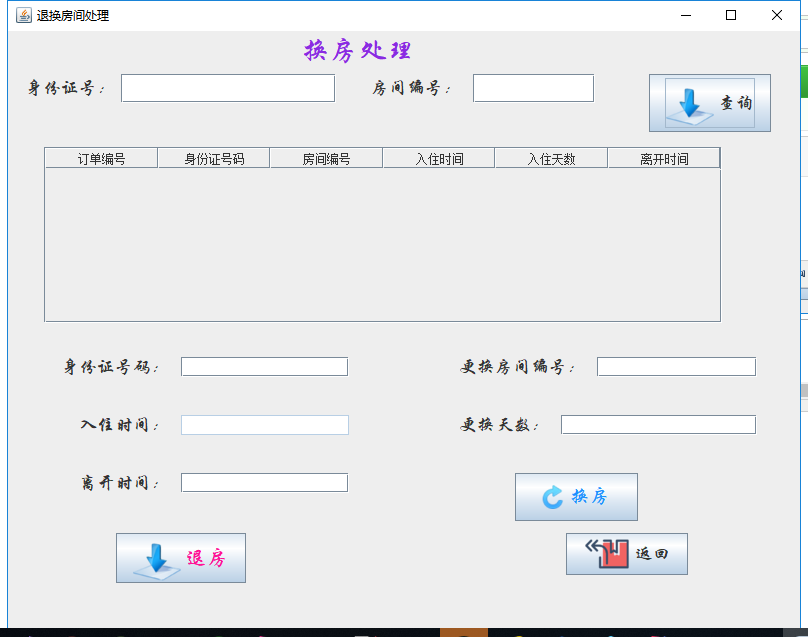
【核心代码】
package com.sqc.view;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.border.EmptyBorder;
import com.sqc.dao.UserDao;
import com.sqc.model.User;
import com.sqc.util.DbUtil;
import com.sqc.util.StringUtil;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.BoxLayout;
import java.awt.FlowLayout;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.ImageIcon;
import javax.swing.JTextField;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.awt.event.ActionEvent;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
public class LoginFra extends JFrame {
private JPanel contentPane;
private JTextField userName_text;
private JPasswordField password_text;
private DbUtil dbUtil=new DbUtil();
private UserDao userDao=new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
LoginFra frame = new LoginFra();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public LoginFra() {
setTitle("\u6854\u5B50\u9152\u5E97\u7BA1\u7406\u7CFB\u7EDF");
setResizable(false);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 557, 444);
cOntentPane= new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel password = new JLabel("\u5458\u5DE5\u53F7\uFF1A");
password.setFont(new Font("华文行楷", Font.PLAIN, 18));
password.setIcon(new ImageIcon(LoginFra.class.getResource("/image/head.png")));
JLabel label = new JLabel("\u5BC6 \u7801\uFF1A");
label.setFont(new Font("华文行楷", Font.PLAIN, 18));
label.setIcon(new ImageIcon(LoginFra.class.getResource("/image/password.png")));
userName_text = new JTextField();
userName_text.setColumns(10);
JButton btn_login = new JButton("\u767B \u5F55");
btn_login.setIcon(new ImageIcon(LoginFra.class.getResource("/image/accept.png")));
btn_login.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
loginActionPerformed(e);
}
});
btn_login.setFont(new Font("微软雅黑", Font.PLAIN, 18));
JButton btn_cancel = new JButton("\u6E05 \u9664");
btn_cancel.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
btn_cancelActionPerformed(e);
}
});
btn_cancel.setIcon(new ImageIcon(LoginFra.class.getResource("/image/refersh.png")));
btn_cancel.setFont(new Font("微软雅黑", Font.PLAIN, 18));
password_text = new JPasswordField();
JButton btnNewButton = new JButton("");
btnNewButton.setIcon(new ImageIcon(LoginFra.class.getResource("/image/theme.jpg")));
GroupLayout gl_cOntentPane= new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(69)
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING)
.addComponent(label)
.addComponent(password))
.addGap(39)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(btnNewButton, GroupLayout.PREFERRED_SIZE, 148, GroupLayout.PREFERRED_SIZE)
.addComponent(userName_text, GroupLayout.DEFAULT_SIZE, 202, Short.MAX_VALUE)
.addComponent(password_text))
.addContainerGap(127, Short.MAX_VALUE))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(93)
.addComponent(btn_login)
.addPreferredGap(ComponentPlacement.RELATED, 79, Short.MAX_VALUE)
.addComponent(btn_cancel, GroupLayout.PREFERRED_SIZE, 151, GroupLayout.PREFERRED_SIZE)
.addGap(67))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(33)
.addComponent(btnNewButton, GroupLayout.PREFERRED_SIZE, 134, GroupLayout.PREFERRED_SIZE)
.addGap(27)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(password)
.addComponent(userName_text, GroupLayout.PREFERRED_SIZE, 37, GroupLayout.PREFERRED_SIZE))
.addGap(40)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label)
.addComponent(password_text, GroupLayout.PREFERRED_SIZE, 37, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(ComponentPlacement.RELATED, 21, Short.MAX_VALUE)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(btn_login)
.addComponent(btn_cancel, GroupLayout.PREFERRED_SIZE, 54, GroupLayout.PREFERRED_SIZE))
.addGap(37))
);
contentPane.setLayout(gl_contentPane);
}
/**
* 登录事件处理
* @param e
*/
private void loginActionPerformed(ActionEvent evt) {
String userName=this.userName_text.getText();
String password=new String(this.password_text.getPassword());
if(StringUtil.isEmpty(userName)){
JOptionPane.showMessageDialog(null, "用户名不能为空!");
return;
}
if(StringUtil.isEmpty(password)){
JOptionPane.showMessageDialog(null, "密码不能为空!");
return;
}
User user=new User(userName,password);
Connection con=null;
try {
con=dbUtil.getCon();
User currentUser=userDao.login(con, user);
if(currentUser!=null){
dispose();
new MainFra().setVisible(true);
}else{
JOptionPane.showMessageDialog(null, "用户名或者密码错误!");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
try {
dbUtil.closeCon(con);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
/**
* 重置事件处理
* @param e
*/
private void btn_cancelActionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
this.userName_text.setText("");
this.password_text.setText("");
}
}