作者:采臣--小青在这_203 | 来源:互联网 | 2023-08-23 12:26
1.在application.properties里面添加连接数据库的信息。spring.datasource.urljdbc:mysql:localhost:3306empdb?
1.在application.properties里面添加连接数据库的信息。
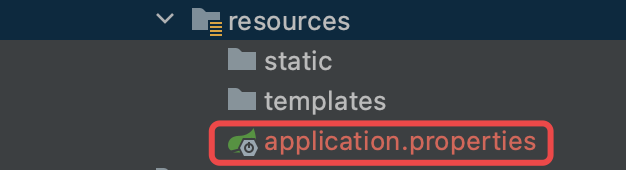
spring.datasource.url=jdbc:mysql://localhost:3306/empdb?characterEncoding=utf8&serverTimezone=Asia/Shanghai&useSSL=false
spring.datasource.username=root
spring.datasource.password= //写自己的数据库密码
2.创建Emp实体类,因为Mybatis框架是通过实体类和表之间的对应关系生成JDBC代码的,所以必须要有实体类。
public class Emp {private Integer id;private String name;private Integer sal;private String job;@Overridepublic String toString() {return "Emp{" +"id=" + id +", name='" + name + '\'' +", sal=" + sal +", job='" + job + '\'' +'}';}public Integer getId() {return id;}public void setId(Integer id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public Integer getSal() {return sal;}public void setSal(Integer sal) {this.sal = sal;}public String getJob() {return job;}public void setJob(String job) {this.job = job;}
}
3.创建EmpMapper,Mybatis框架需要从EmpMapper接口中得到需要执行的SQL语句,以及对象和表之间的映射关系。在Mapper中添加@Mapper注解。
- @Insert注解修饰一个insert方法。
- @Select是查询的注解,返回值为集合,Mybatis框架生成的jdbc代码会将查询到的数据封装到Emp对象中,然后把Emp对象装进list集合,通过方法返回。
import entity.Emp;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;/*
* @Mapper
* 将接口交给Spring管理,不用再写pom.xml文件,为此接口生成一个实现类让其他类引用。
* */
@Mapper
public interface EmpMapper {@Insert("insert into myemp values(name,#{name},#{sal},#{job})")void insert(Emp emp);@Select("select * from myemp")List select();//查询带条件而且查询结果是一个对象时@Select("select * from myemp where id=#{id}")Emp selectById(int id);//查询到结果有多条数据,用一个对象作为返回会报错,应该用集合@Select("select * from myemp where name=#{name}")List selectByName(String name);//方法返回值为int时 返回的是生效的行数@Update("update myemp set name=#{name},sal=#{sal},job=#{job} where id=#{id}")int update(Emp emp);//删除注解@Delete("delete from myemp where id=#{id}")void deleteById(int id);
}
4.单元测试,在test目录下。
import cn.tedu.boot31_1.entity.Emp;
import cn.tedu.boot31_1.mapper.EmpMapper;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;@SpringBootTest
class Boot311ApplicationTests {//把Mapper装配起来@Autowired(required = false)EmpMapper mapper;@Testvoid contextLoads() {Emp e = new Emp();e.setName("曹操");e.setSal(10000);e.setJob("司空");mapper.insert(e);}@Testvoid select(){List list = mapper.select();for (Emp e:list){System.out.println(e.getName()+":"+e.getSal()+":"+e.getJob());}}@Testvoid select02(){Emp e = mapper.selectById(3);System.out.println(e);}@Testvoid select03() {List list = mapper.selectByName("诸葛亮");System.out.println(list);}@Testvoid update(){Emp e = new Emp();e.setId(4);e.setName("卧龙");e.setSal(5000);e.setJob("程序员");int row = mapper.update(e);System.out.println("行数="+row);}@Testvoid delete(){mapper.deleteById(4);}
}
添加成功
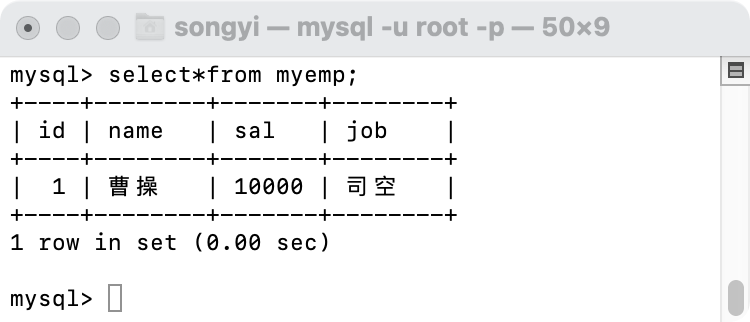
查询成功
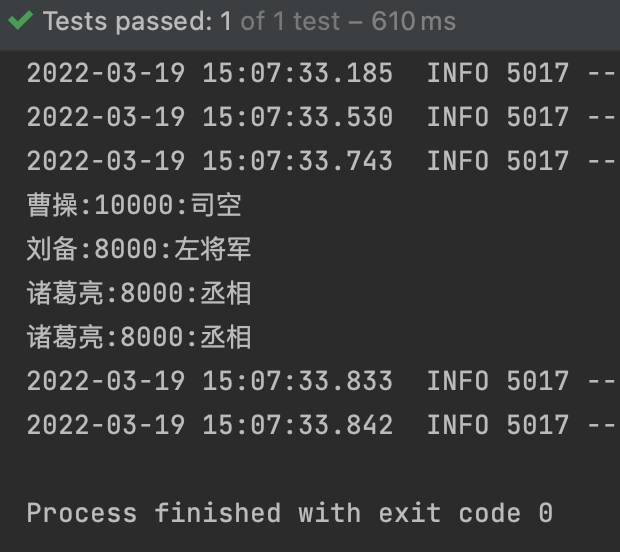
查询id为3的
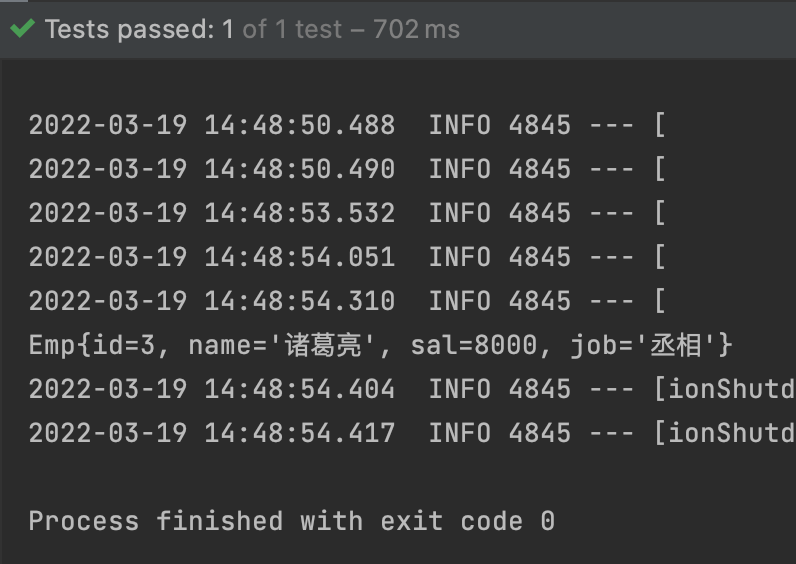
查询两个诸葛亮
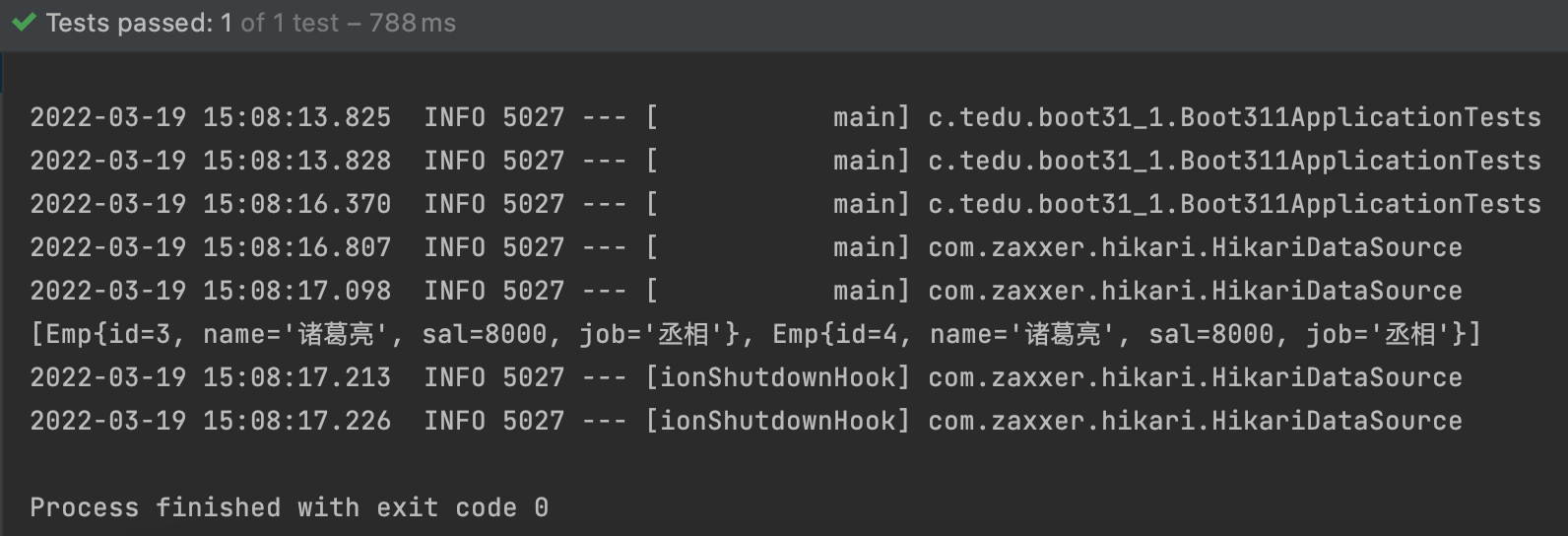
修改id为4的诸葛亮信息并显示修改的条数
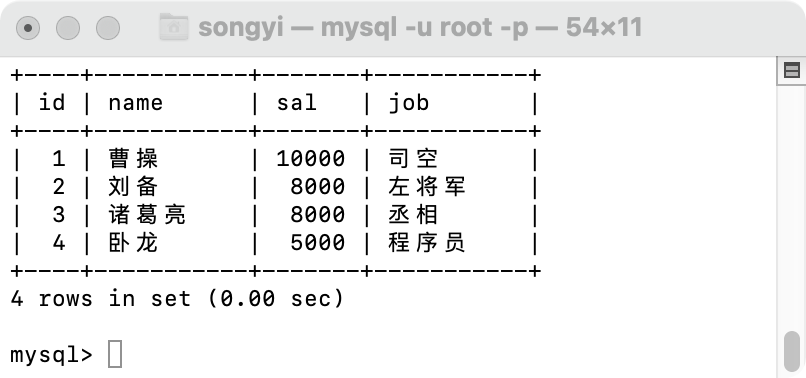
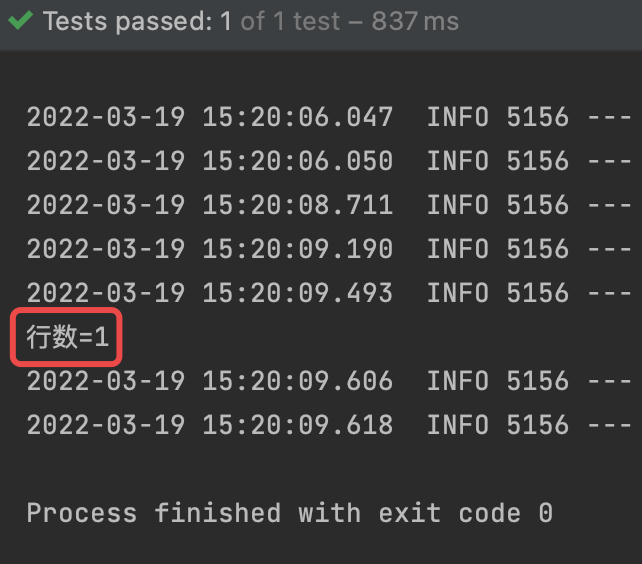
删除四号员工
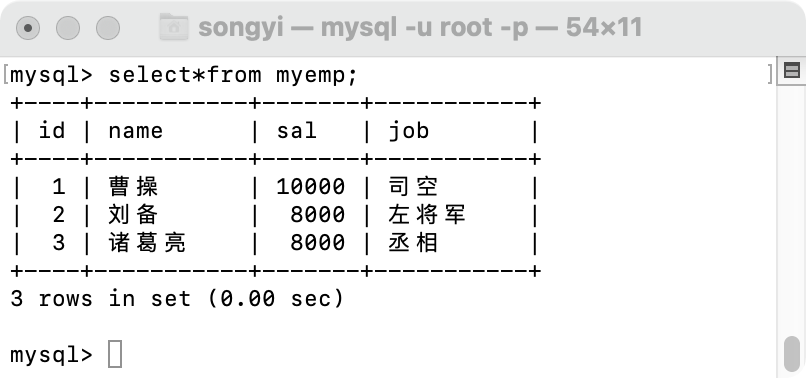