大部分情况下爬取的数据特别灵活,不一定只有指定的几个字段数据,这时候就需要将数据存储在非关系型数据库中了,MongoDB 是由C++语言编写的,是一个基于分布式文件存储的开源数据库系统。在高负载的情况下,添加更多的节点,可以保证服务器性能。MongoDB 旨在为WEB应用提供可扩展的高性能数据存储解决方案。MongoDB 将数据存储为一个文档,数据结构由键值(key=>value)对组成。MongoDB 文档类似于 JSON 对象。字段值可以包含其他文档,数组及文档数组。

[来源:菜鸟教程]
连接MongoDB
我们可以使用pymongo库连接数据库,使用pip3 install pymongo
安装。
import pymongoclient = pymongo.MongoClient(host='127.0.0.1',port=27017)
#client = pymongo.MongoClient(mongodb://127.0.0.1:27017/)第二中方式连接数据库
我自己的MongoDB没有设置用户名和密码,不需要进行认证。如果你连接MongoDB需要认证请查阅:http://api.mongodb.com/python/current/examples/authentication.html。特别注意mongoDB版本导致的认证机制不同,否则认证失败。
选择数据库
和MySql不同,MySql在连接时需要知道数据库,而mongodb可以不需要,连接成功后指定即可,方式如下:
import pymongoclient = pymongo.MongoClient(host='127.0.0.1',port=27017)#连接数据库
db = client.pymongoTest#指定pymongoTest数据库,也可使用db = client['pymongoTest']
选择集合
选定数据库后我们再选定集合,从而可以将文档内容插入集合中。
import pymongoclient = pymongo.MongoClient(host='127.0.0.1',port=27017)
db = client.pymongoTest#指定pymongoTest数据库
collection = db.person#指定person集合,也可使用collection = db['person']
#指定的集合可以不存在,当插入数据后生成。
数据插入
我们可以使用insert_one()
和 insert_many()
分别插入单条记录和多条记录。
p1 = {'_id':1,'name':'lisi','age':20,'addr':'xian'
}
p2 = {'name':'Jake','age':30,'addr':'beijing'
}
p3 = {'name':'Tom','age':25,'addr':'shanghai'
}
#不写_id会自动创建_id值result = collection.insert_one(p1)
print(type(result))
print(result.inserted_id)
print('=========================================================')
result = collection.insert_many([p2,p3])#插入多个数据用列表表示
print(type(result))
print(result.inserted_ids)
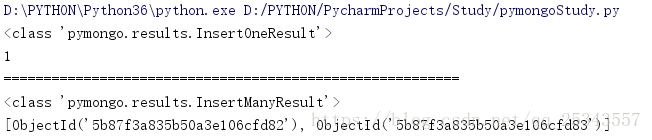
insert_one()
方法返回InsertOneResult对象 ,调用其inserted_id属性获取文档的_id
属性。insert_many()
方法返回InsertManyResult对象 ,调用其inserted_ids属性获取文档的_id
属性。注意多了个s
。
数据查询
我们可以使用find_one()
和find()
方法进行查询。前者查询单条数据,返回字典类型,后者返回一个生成器对象。
查询name为Jake的数据:
result = collection.find_one({'name':'Jake'})
print(result)

根据_id查询:
由于文档中的_id类型是ObjectId类型,所以我们需要导入bson中的ObjectId。
from bson.objectid import ObjectIdresult = collection.find_one({'_id':ObjectId("5b87f3a835b50a3e106cfd83")})
print(result)

查询addr为‘xian’的数据:
results = collection.find({'addr':'xian'})
for result in results:print(result)

查询age大于20的数据:
results = collection.find({'age':{'$gt':20}})
for result in results:print(result)

比较查询的值不是单纯的数值,而是一个字典,key为比较条件(大于或等于,等等),value为具体的数值。诸如$gt
这样的比较符号如下:
- (>) 大于 - $gt
- (<) 小于 - $lt
- (>&#61;) 大于等于 - $gte
- (<&#61; ) 小于等于 - $lte
- (!&#61; )不等于 - $ne
- 在范围内 - $in
- 不在范围内 - $nin
除了比较符号还要其他符号见mongodb官方文档&#xff1a;https://docs.mongodb.com/manual/reference/operator/query/。
数据更新
我们可以使用update_one()
和update_many()
方法更新数据。前者更新符合条件的第一条数据&#xff0c;后者将所有符合条件的数据都更新。
condition &#61; {&#39;name&#39;:&#39;Lucy&#39;}
result &#61; collection.update_one(condition,{&#39;$set&#39;:{&#39;age&#39;:30}})#将name为Lucy的数据中的age更新为30
print(result)
print(result.matched_count)#符合条件的条目数
print(result.modified_count)#影响的条目数

不使用$set
&#xff0c;使用$inc
将age加1。
condition &#61; {&#39;name&#39;:&#39;Lucy&#39;}
result &#61; collection.update_one(condition,{&#39;$inc&#39;:{&#39;age&#39;:1}})
print(result)
print(result.matched_count)
print(result.modified_count)
使用update_many()
更新多条数据&#xff0c;将年龄大于等于18的人age属性加1。
condition &#61; {&#39;age&#39;:{&#39;$gte&#39;:18}}
result &#61; collection.update_many(condition,{&#39;$inc&#39;:{&#39;age&#39;:1}})
print(result)
print(result.matched_count)
print(result.modified_count)

符合条件的条目数为5条&#xff0c;影响了5条。
数据删除
我们可以使用delete_one()
和delete_many()
删除数据&#xff0c;前者删除一条数据&#xff0c;后者删除多条数据。
#删除name为Jake的数据
result &#61; collection.delete_one({&#39;name&#39;:&#39;Jake&#39;})
print(result)
print(result.deleted_count)
#删除age大于20的数据
result &#61; collection.delete_many({&#39;age&#39;:{&#39;$gt&#39;:20}})
print(result)
print(result.deleted_count)

其他方法
数据统计
查询结果调用&#96;count()&#96; 方法可以统计出共有多少条数据。
result &#61; collection.find().count()
print(&#39;总条目为&#xff1a;&#39;,result)

数据排序
查询结果调用&#96;sort()&#96; 方法可以将数据按照某一字段设置升序或降序排序。
result &#61; collection.find().sort(&#39;age&#39;,pymongo.ASCENDING)#按照age升序排序
for item in result:print(item)
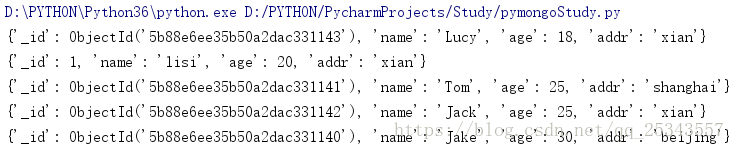
偏移
有时候我们不想取前几条数据&#xff0c;我们可以使用&#96;skip()&#96; 设置偏移&#xff0c;忽略前面的数据。
result &#61; collection.find().sort(&#39;age&#39;,pymongo.ASCENDING).skip(2)#偏移量为2&#xff0c;获取第三条数据后的数据
for item in result:print(item)
设置偏移前&#xff1a;
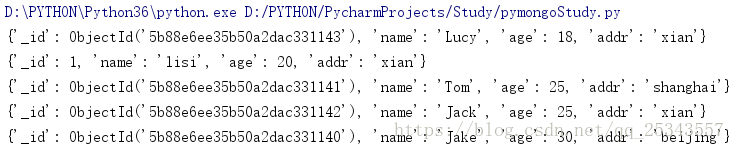
设置偏移后&#xff1a;

我们还可以使用limit()
限制返回结果的数目。
result &#61; collection.find().sort(&#39;age&#39;,pymongo.ASCENDING).limit(2)#只获取2条数据
for item in result:print(item)
限制前&#xff1a;
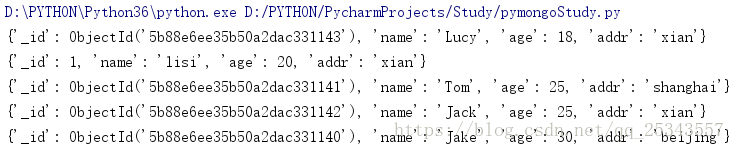
限制后&#xff1a;
