作者: | 来源:互联网 | 2023-09-04 21:50
图片一用内部存储实现文件写入和读取功能packagecom.example.wl1;importandroidx.appcompat.app.AppCompatActivity;i
图片一 用内部存储实现文件写入和读取功能
package com.example.wl1;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
findViewById(R.id.bt_xieru);
findViewById(R.id.bt_duqu);
EditText editText1=findViewById(R.id.et_xieru);
EditText editText2=findViewById(R.id.et_duqu);
}
public void click1(View view){
EditText editText1=findViewById(R.id.et_xieru);
EditText editText2=findViewById(R.id.et_duqu);
String fileName="data.txt";
String context=editText1.getText().toString();
FileOutputStream fos=null;
try {
fos=openFileOutput(fileName,MODE_PRIVATE);
fos.write(context.getBytes());
}catch(Exception e){
e.printStackTrace();
}finally {
if (fos!=null){
try {
fos.close();
}catch(IOException e){
e.printStackTrace();
}
}
}
Toast.makeText(this,"保存成功",Toast.LENGTH_SHORT).show();
}
public void click2(View view){
EditText editText2=findViewById(R.id.et_duqu);
String context="";
FileInputStream fis=null;
try {
fis=openFileInput("data.txt");
byte[]buffer=new byte[fis.available()];
fis.read(buffer);
context=new String(buffer);
}catch (Exception e){
e.printStackTrace();
}finally {
if (fis!=null){
try{
fis.close();
}catch(IOException e){
e.printStackTrace();
}
}
}
editText2.setText(context);
}
}
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/et_xieru"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入你想写入的内容"
android:textSize="30dp"
android:layout_marginTop="20dp"/>
<Button
android:id="@+id/bt_xieru"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="click1"
android:text="写入"
android:textSize="30dp"
android:layout_marginLeft="20dp" />
<EditText
android:id="@+id/et_duqu"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="显示读取的内容"
android:textSize="30dp"
android:layout_marginTop="20dp"/>
<Button
android:id="@+id/bt_duqu"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="click2"
android:text="读取"
android:textSize="30dp"
android:layout_marginLeft="20dp" />
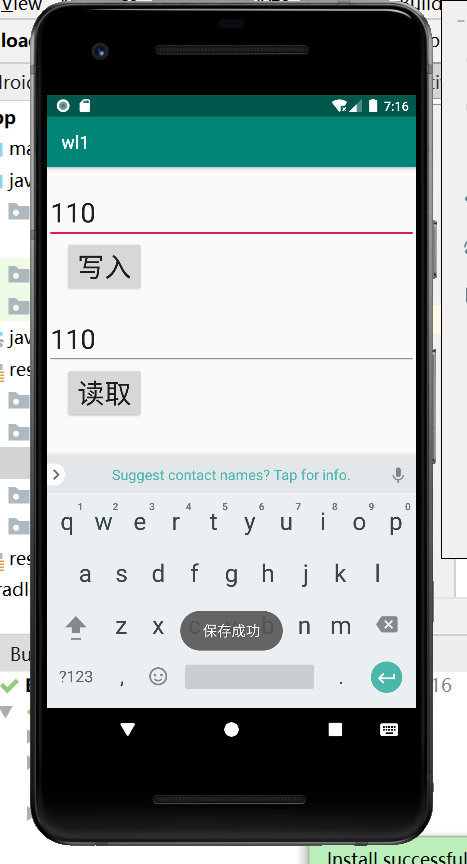
2. 使用sharedpreference实现记住密码功能
package com.example.wl1;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.SharedPreferences;
import java.util.HashMap;
import java.util.Map;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.EditText;
import android.widget.Toast;
import java.util.Map;
public class MainActivity extends AppCompatActivity implements android.view.View.OnClickListener, CompoundButton.OnCheckedChangeListener {
private EditText et_account;
private EditText et_password;
private Button btn_login;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
Map userInfo=saveQQ.getUserInfo(this);
if(userInfo!=null){
et_account.setText(userInfo.get("account"));
et_password.setText(userInfo.get("password"));
}
}
private void initView() {
et_account=findViewById(R.id.et_1);
et_password=findViewById(R.id.et_2);
btn_login=findViewById(R.id.btn_1);
btn_login.setOnClickListener(this);
CheckBox cb1=findViewById(R.id.cb_1);
cb1.setOnCheckedChangeListener(this);
CheckBox cb2=findViewById(R.id.cb_2);
cb2.setOnCheckedChangeListener(this);
}
@Override
public void onClick(View view) {
switch (view.getId()){
case R.id.btn_1:
String account=et_account.getText().toString().trim();
String password=et_password.getText().toString();
if(TextUtils.isEmpty(account)){
Toast.makeText(this,"请输入账号",Toast.LENGTH_LONG).show();
return;
}
if(TextUtils.isEmpty(password)){
Toast.makeText(this,"请输入密码",Toast.LENGTH_LONG).show();
return;
}
Toast.makeText(this,"登录成功",Toast.LENGTH_LONG).show();
}
}
public static class saveQQ {
public static Map getUserInfo(Context context) {
SharedPreferences sp = context.getSharedPreferences("data", context.MODE_PRIVATE);
String account = sp.getString("Username", null);
String password = sp.getString("pwd", null);
MapuserMap=new HashMap();
userMap.put("account", account);
userMap.put("password", password);
return userMap;
}
public static boolean saveUserInfo(Context context, String account, String password) {
SharedPreferences sp=context.getSharedPreferences("data",context.MODE_PRIVATE);
SharedPreferences.Editor editor=sp.edit();
editor.putString("Username",account);
editor.putString("pwd",password);
editor.commit();
return true;
}
}
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
switch (compoundButton.getId()){
case R.id.cb_1:
String account=et_account.getText().toString().trim();
String password=et_password.getText().toString();
boolean isSaveSuccess=saveQQ.saveUserInfo(this,account,password);
if(isSaveSuccess&&b){
Toast.makeText(this,"保存成功",Toast.LENGTH_LONG).show();
}else {
Toast.makeText(this,"保存失败",Toast.LENGTH_LONG).show();
et_account.setText(null);
et_password.setText(null);
}
break;
case R.id.cb_2:
account=et_account.getText().toString().trim();
password=et_password.getText().toString();
if(TextUtils.isEmpty(account)){
Toast.makeText(this,"请输入账号",Toast.LENGTH_LONG).show();
return;
}
if(TextUtils.isEmpty(password)){
Toast.makeText(this,"请输入密码",Toast.LENGTH_LONG).show();
return;
}
if(b)
Toast.makeText(this,"登录成功",Toast.LENGTH_LONG).show();
else {
Toast.makeText(this,"请登录",Toast.LENGTH_LONG).show();
}
}
}
}
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FADFE9"
tools:context=".MainActivity">
<TextView
android:id="@+id/tv_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="账号"
android:textColor="#000000"
android:textSize="20sp"
android:layout_marginTop="30dp"
android:layout_marginLeft="20dp"
android:padding="10dp"/>
<EditText
android:id="@+id/et_1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入用户名"
android:layout_toRightOf="@id/tv_1"
android:layout_marginTop="30dp"
android:padding="10dp"
android:textSize="20sp"/>
<TextView
android:id="@+id/tv_2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="密码"
android:textColor="#000000"
android:textSize="20sp"
android:layout_marginTop="30dp"
android:layout_marginLeft="20dp"
android:layout_below="@id/tv_1"
android:padding="10dp"/>
<EditText
android:id="@+id/et_2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入密码"
android:layout_toRightOf="@id/tv_2"
android:layout_below="@id/et_1"
android:layout_marginTop="30dp"
android:padding="10dp"
android:textSize="20sp"/>
<CheckBox
android:id="@+id/cb_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="记住密码"
android:textColor="#000000"
android:textSize="18sp"
android:layout_below="@id/tv_2"
android:padding="5dp"
android:layout_marginTop="30dp"
android:layout_marginLeft="20dp"/>
<CheckBox
android:id="@+id/cb_2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="自动登录"
android:textColor="#000000"
android:textSize="18sp"
android:layout_below="@id/tv_2"
android:layout_toRightOf="@id/cb_1"
android:padding="5dp"
android:layout_marginTop="30dp"
android:layout_marginLeft="20dp"/>
<Button
android:id="@+id/btn_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="登录"
android:textColor="#000000"
android:textSize="18sp"
android:layout_below="@id/tv_2"
android:padding="5dp"
android:layout_marginTop="25dp"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@id/cb_2"/>
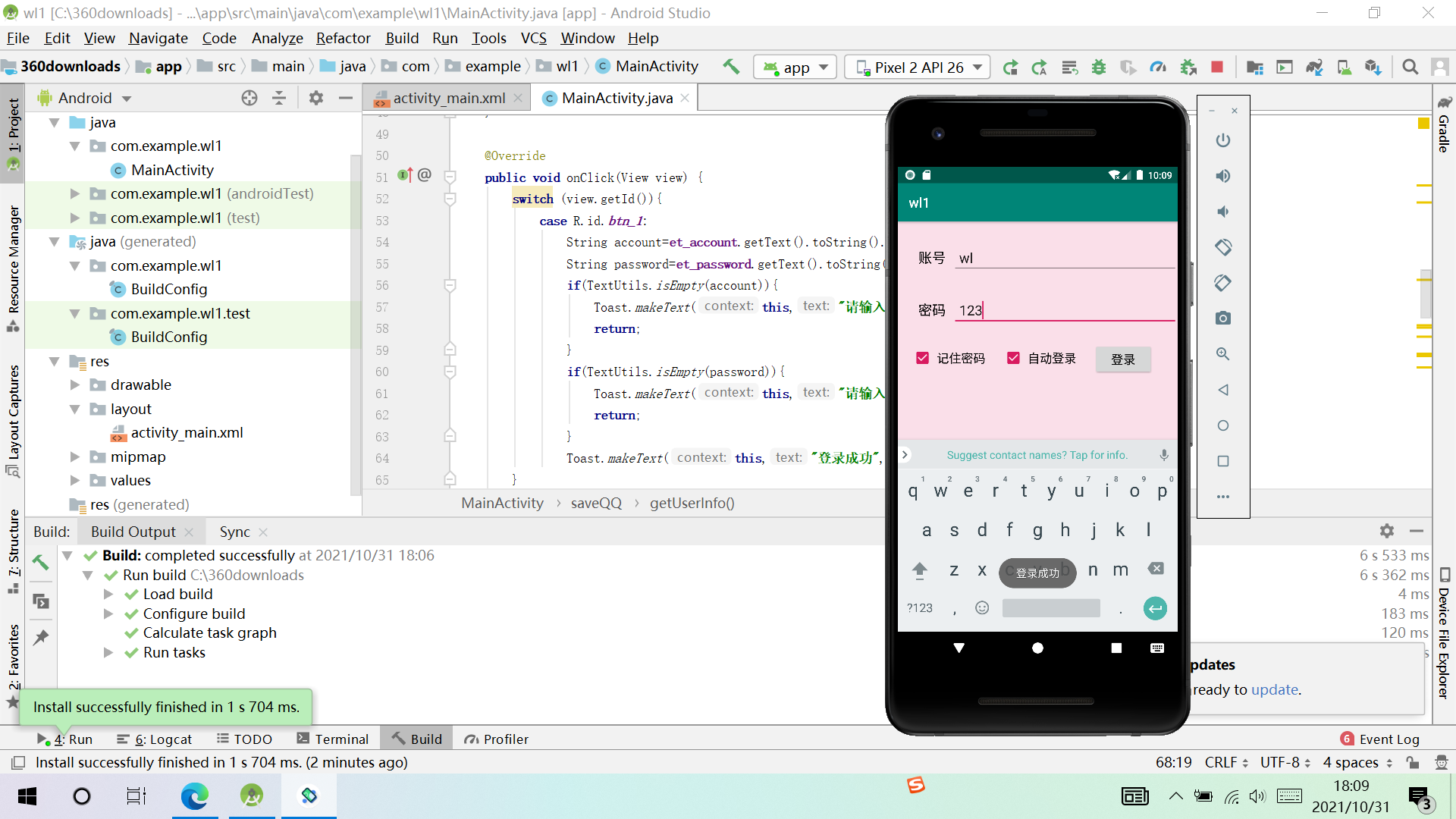