
6、iOS 与 Android dom 结构的区别
dom 属性和节点结构类似
名字和属性命名不同
android 的 resourceid 和 ios 的 name
android 的 content-desc 和 ios 的 accessibility-id
7、定位方法
测试步骤三要素
定位、交互、断言
定位方式:
id 定位
accessibilty_id 定位
xpath 定位
classname 定位(不推荐)
三、常见的控件定位方法
Accessibility ID
:识别一个唯一的 UI 元素,
对于 XCUITest 引擎,它对应的的属性名是 accessibility-id,
对于 Android 系统的页面元素,对应的属性名是 content-desc
Class name
:
对于 iOS 系统,它的 class 属性对应的属性值会以XCUIElementType开头,
对于 Android 系统,它对应的是 UIAutomator2 的 class 属性(e.g.: android.widget.TextView)
ID
:原生元素的标识符,
Android 系统对应的属性名为resource-id,
iOS 为name
Name
:元素的名称
XPath
:使用 xpath 表达式查找页面所对应的 xml 的路径
1、App 定位方式进阶
Image
:通过匹配 base 64 编码的图像文件定位元素
Android UiAutomator (UiAutomator2 only)
:使用 UI Automator 提供的 API, 尤其是 UiSelector 类来定位元素,在 Appium 中,会发送 Java 代码作为字符串发送到服务器,服务器在应用程序的环境中执行这段代码,并返回一个或多个元素
Android View Tag (Espresso only)
:使用 view tag 定位元素
Android Data Matcher (Espresso only)
:使用 Espresso 数据匹配器定位元素
IOS UIAutomation:
在 iOS 应用程序自动化时,可以使用苹果的 instruments 框架查找元素
2、选择定位器通用原则
与研发约定的属性优先
android 推荐 content-description
ios 推荐 label
身份属性 id
组合定位 xpath,css
其它定位
3、元素定位的写法
返回单个元素 WebElement
driver.find_element(AppiumBy.xxx, "xxx属性值")
返回元素列表 [WebElement, WebElement, WebElement…]
driver.find_elements(AppiumBy.xxx, "xxx属性值")
driver.find_element(AppiumBy.ID, “ID属性值”)
driver.find_element(AppiumBy.XPATH, “xpath表达式”)
driver.find_element(AppiumBy.CLASS_NAME, “CLASS属性值”)
driver.find_element(AppiumBy.ACCESSIBILITY_ID, “ACCESSIBILITY_ID表达式”)
driver.find_element(AppiumBy.ANDROID_UIAUTOMATOR, “android uiautomator 表达式”)
driver.find_element(AppiumBy.IOS_UIAUTOMATION, “ios uiautomation 表达式”)
driver.find_element(AppiumBy.ANDROID_VIEWTAG, “ESPRESSO viewtag 表达式”)
driver.find_element(AppiumBy.ANDROID_DATA_MATCHER, “ESPRESSO data matcher 表达式”)
driver.find_element(AppiumBy.IMAGE, “IMAGE图片”)
driver.find_elements(AppiumBy.ID, “ID属性值”)
driver.find_elements(AppiumBy.XPATH, “xpath表达式”)
driver.find_elements(AppiumBy.CLASS_NAME, “CLASS属性值”)
driver.find_elements(AppiumBy.ACCESSIBILITY_ID, “ACCESSIBILITY_ID表达式”)
driver.find_elements(AppiumBy.ANDROID_UIAUTOMATOR, “android uiautomator 表达式”)
driver.find_elements(AppiumBy.IOS_UIAUTOMATION, “ios uiautomation 表达式”)
driver.find_elements(AppiumBy.ANDROID_VIEWTAG, “ESPRESSO viewtag 表达式”)
driver.find_elements(AppiumBy.ANDROID_DATA_MATCHER, “ESPRESSO data matcher 表达式”)
driver.find_elements(AppiumBy.IMAGE, “IMAGE图片”)
4、ID 定位
通过身份标识 id 查找元素
写法:find_element(AppiumBy.ID, “ID属性值”)
self.driver.find_element(AppiumBy.ID,"android:id/text1")
通过身份标识 id 查找元素,对应的是resource-id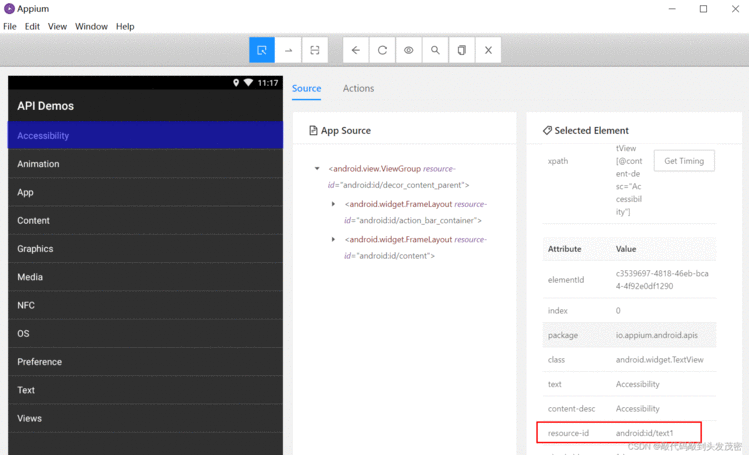
5、ACCESSIBILITY_ID 定位
通过 accessibility id 查找元素
写法:find_element(AppiumBy.ACCESSIBILITY_ID, "ACCESSIBILITY_ID属性值")
self.driver.find_element(AppiumBy.ACCESSIBILITY_ID,'App')
通过 accessibility_id查找元素,对应的是content-desc
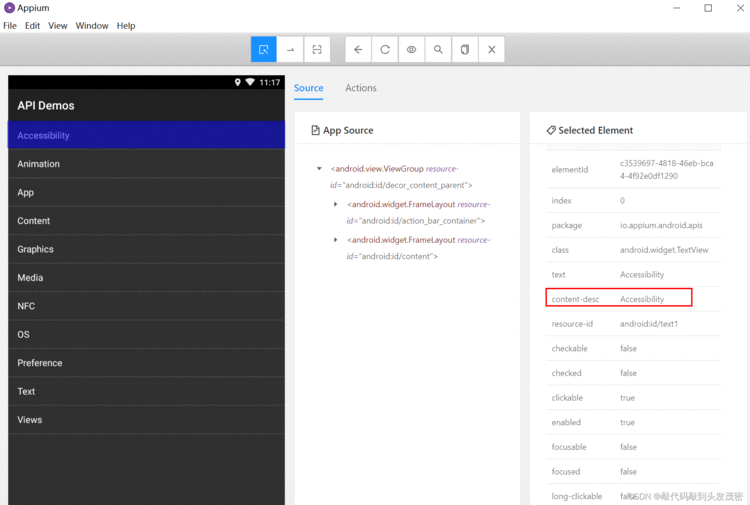
6、XPath 定位
/
从根节点选取(取子节点)。
//
从匹配选择的当前节点选择文档中的节点,而不考虑它们的位置(取子孙节点)
.
选取当前节点。
..
选取当前节点的父节点。
@
选取属性。
a、XPath 单属性定位
基本表达式://*[@属性名=‘属性值’]
self.driver.find_element(AppiumBy.XPATH,'//*[@text="Content"]')
b、XPath 多属性定位
表达式://*[@属性名=‘属性值’ and @属性名=‘属性值’ ]
7、Android 原生定位
元素属性定位
ID 定位
文本定位
文本匹配定位
父子关系定位
兄弟关系定位
a、Android 原生定位 - 单属性定位
格式 &#39;new UiSelector().属性名("<属性值>")&#39;
比如&#xff1a;‘new UiSelector().resourceId(“android:id/text1”)’
注意外面是单引号&#xff0c;里面是双引号&#xff0c;顺序不能变
可以简写为 属性名(“<属性值>”)’
比如&#xff1a;·resourceId(“android:id/text1”)
def test_uiautomator(self):"""android uiautomator"""element&#61;self.driver.find_element(AppiumBy.ANDROID_UIAUTOMATOR,&#39;new UiSelector().resourceId("android:id/text1")&#39;)element.click()
b、Android 原生定位-组合定位
多个属性同时确定元素的&#xff08;多个属性任意组合 &#xff0c;不限长度&#xff09;
def test_uiautomator1(self):"""android uiautomator"""element&#61;self.driver.find_element(AppiumBy.ANDROID_UIAUTOMATOR,&#39;new UiSelector().resourceId("android:id/text1").text("App")&#39;)element.click()
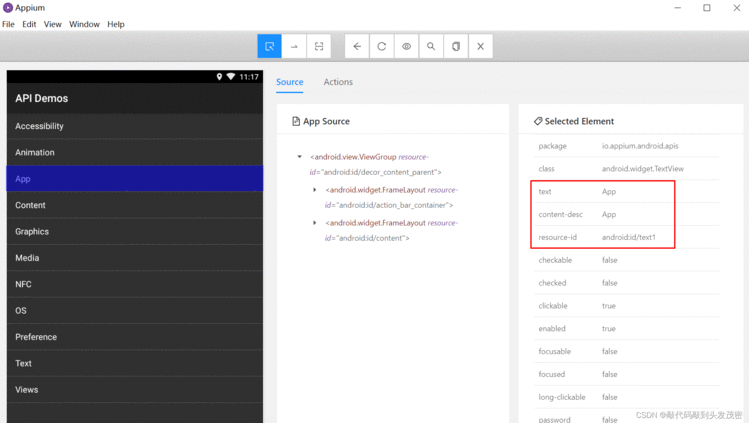
c、Android 原生定位-模糊匹配
文字包含
文字以 x 开头
文字正则匹配
def test_android_uiautomator_by_text_contains(self):print(self.driver.find_element(AppiumBy.ANDROID_UIAUTOMATOR, &#39;new UiSelector().textContains("ssi")&#39;).text)def test_android_uiautomator_by_text_start_with(self):print(self.driver.find_element(AppiumBy.ANDROID_UIAUTOMATOR, &#39;new UiSelector().textStartsWith("Ani")&#39;).text)def test_android_uiautomator_by_text_match(self):print(self.driver.find_element(AppiumBy.ANDROID_UIAUTOMATOR, &#39;new UiSelector().textMatches("^Pre.*")&#39;).text)
d、Android 原生定位-层级定位
兄弟元素定位 fromParent
父子结点定位 childSelector, 可以传入 resourceId() , description() 等方法
new UiSelector().text("App").fromParent(text("Text"))
new UiSelector().className("android.widget.ListView").childSelector(text("Text"))
滑动查找元素
new UiScrollable(new UiSelector().scrollable(true).instance(0)).scrollIntoView(new UiSelector().text("查找的元素文本").instance(0))
e、滑动查找元素
格式如下&#xff1a;
‘new UiScrollable(new
UiSelector().scrollable(true).instance(0)).scrolllntoView(new
UiSelector().text("查找的文本”).instance(0));&#39;