翻译自:http://www.appcoda.com/ios-programming-send-sms-text-message
Message UI框架不仅可以来发送email,它也为开发者提供了在app中发送短息的界面。使用MFMailComposeViewController类来处理email,使用MFMessageComposeViewController来处理短信。
使用MFMessageComposeViewController与使用邮件很类似。如果你阅读过sending email 和 creating email with attachment,这两篇教程的话,使用这个不过小菜一碟。总之,我们将会通过一个简单地例子,来展示如实使用MFMessageComposeViewController这个类。
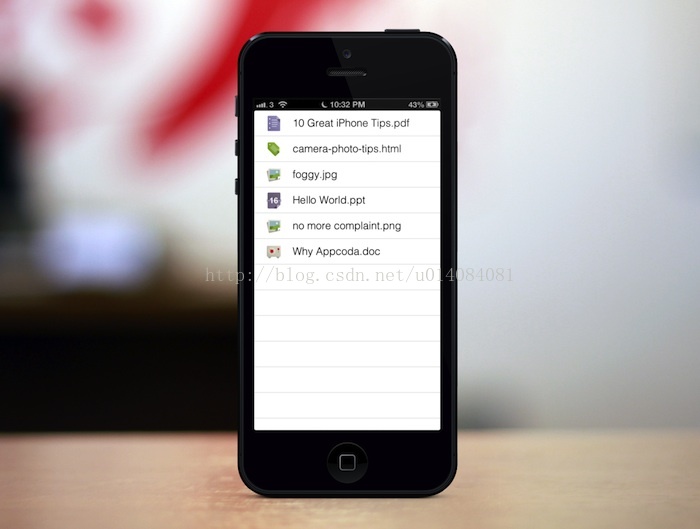
Demo App 我们将会使用上一次的demo app,但是会更改一下。app仍然显示一个文件列表。然而,当用户点击table view的某一行的时候,app将会跳转到对应的信息界面,并附带上短信类容。
Getting Started 在这个地方下载项目模板 download the project template ,已经创建好Storyboard,加载好tableview。
Importing the MessageUI Framework 第一步,导入Message UI框架。
Implementing the Delegate 在“AttachmentTableViewController.m”文件中,加入如下的代码:导入Message UI头文件,并遵守MFMessageComposeViewControllerDelegate协议。
#import @interface AttachmentTableViewController ()
MFMessageComposeViewControllerDelegate协议定义了一个方法,该方法在短信结束时调用。实现此方法,并处理各种不同的状况。
- 用户取消编辑SMS
- 用户点击了发送按钮,SMS发送成功
- 用户点击了发送按钮,SMS发送失败
在“AttachmentTableViewController.m”文件中,加入如下的代码。在这里,我们显示一个alert信息,当出现第3中状况的时候。对于其它的状况,则dimiss掉信息界面。
- (void)messageComposeViewController:(MFMessageComposeViewController *)controller didFinishWithResult:(MessageComposeResult) result
{switch (result) {case MessageComposeResultCancelled:break;case MessageComposeResultFailed:{UIAlertView *warningAlert = [[UIAlertView alloc] initWithTitle:@"Error" message:@"Failed to send SMS!" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];[warningAlert show];break;}case MessageComposeResultSent:break;default:break;}[self dismissViewControllerAnimated:YES completion:nil];
}
Bring Up the Message Composer
当用户点击某一行的时候,来弹出message composer,如下:
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{NSString *selectedFile = [_files objectAtIndex:indexPath.row];[self showSMS:selectedFile];
}
“showSMS:”方法是初始话SMS内容的核心代码,如下:
- (void)showSMS:(NSString*)file {if(![MFMessageComposeViewController canSendText]) {UIAlertView *warningAlert = [[UIAlertView alloc] initWithTitle:@"Error" message:@"Your device doesn't support SMS!" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];[warningAlert show];return;}NSArray *recipents = @[@"12345678", @"72345524"];NSString *message = [NSString stringWithFormat:@"Just sent the %@ file to your email. Please check!", file];MFMessageComposeViewController *messageController = [[MFMessageComposeViewController alloc] init];messageController.messageComposeDelegate = self;[messageController setRecipients:recipents];[messageController setBody:message];// Present message view controller on screen[self presentViewController:messageController animated:YES completion:nil];
}
虽然大部分iOS设备都能发送短信,但作为一个coder,要考虑到异常情况。如果你的app在iPod touch上使用,但iMessage却不支持。在这种情况下,设备明显不能发送短信。所以在代码的开始位置,我们要确定设备是否允许发送短息,使用MFMessageComposeViewController的canSendText方法。
Compile and Run the App 例子简单,运行app在真机上测试,如下:
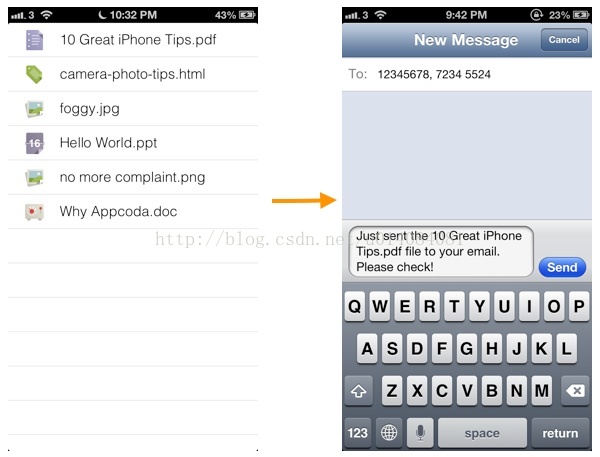
What if You Don’t Want In-App SMS 上面的方法可以在你的app中无缝整合短信功能。但是如果你想直接调用短信app来发送短信,可以使用如下的代码:
[[UIApplication sharedApplication] openURL: @"sms:98765432"];
最终完整的代码:download the full source code here.