-Android初学者对于一种控件是有些许抵触情绪的,那就是ListView。感觉很复杂,又要有子布局,多数又要自定义,很麻烦,可以这么说,ListView是所有控件里面最难的,也是最最常用的。但是,其实你仔细想一下,所有的布局控件都是有迹可循,又有着固定套路的,说白了ListView这个Looper比较大,地球也很大,所以古人很难想想它是圆的,对于新生代程序员来讲,Android开发都是有着深刻套路的,只要你眼界够发散,都能总结。程序开发的目的就是一对多,就像开超市一样。没见哪一个超市售货员只固定卖货给一个人,那得多少售货员。程序员就是另一种形式的售货员。你的产品全在超市里,而且这个超市自给自足。
- 废话不多说,我们来看看最简单的ListView控件使用。
- 第一步、原材料就两个,如下图:

- 第二步、要在布局文件activity_main.xml,书写一个控件ListView,代码如下:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.listview.MainActivity">
<ListView android:id="@+id/list_view" android:layout_width="match_parent" android:layout_height="match_parent" />
LinearLayout>
- 第三步、我们要明白ListView这个控件,多数是要有数据源,子布局,和适配器的。数据源字面就可以理解,我来说说这个子布局,这个就是ListView中每个条目里的样式(一般叫做item)。我们这里用到的数据源是手写的;子布局是Android Studio自带的;适配器是最好用的ArrayAdapter。MainActivity源代码如下:
package com.example.listview;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.ListView;
public class MainActivity extends AppCompatActivity {
private String[] data = {"冰箱","电视","洗衣机","电脑","手机","手电筒","相机","单反","苹果","鸭梨"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ArrayAdapter adapter = new ArrayAdapter<>(
MainActivity.this,android.R.layout.simple_list_item_1,data);
ListView listView = (ListView) findViewById(R.id.list_view);
listView.setAdapter(adapter);
}
}
- 接下来使大招咯,就是我们的自定义ListView的书写,上面的那个实现了以后,你可能觉得很单调,每一个item里面,甚至连个图片都没有,接下来我们自定义做一个。
- 第一步、理论上我们要准备一组图片,分别对应你的每一个item,但是,笔者只是演示,所以全部用ic_launcher了。用到的原材料有五个,如下图:
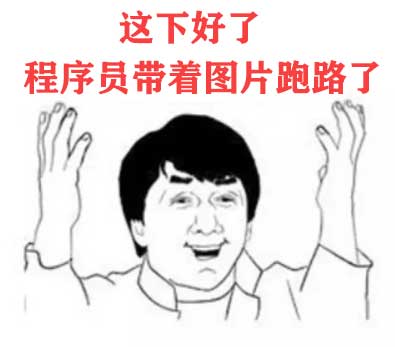
- 第二步、创建一个实体类Things,源代码如下:
package com.example.listview.vo;
/** * 项目名: ViewPager * 包名: com.example.listview.vo * 创建者: Dujiang0311 * 创建时间:2017/4/29 13:27 * 描述: 东西实体类 */
public class Things {
private String name ;
private int imgId;
public Things(String name ,int imgId){
this.name = name;
this.imgId = imgId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getImgId() {
return imgId;
}
public void setImgId(int imgId) {
this.imgId = imgId;
}
}
- 第三步、书写布局文件,things_item.xml文件,代码如下:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent">
<ImageView android:id="@+id/thing_img" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@mipmap/ic_launcher" />
<TextView android:layout_gravity="center_vertical" android:textSize="24dp" android:layout_marginLeft="15dp" android:id="@+id/thing_name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="电冰箱" />
LinearLayout>
- 第四步、接下来才是重头戏,自定义适配器,首先,我们要继承(extends)ArrayAdapter,然后重写其中一个构造方法,接着重写getView方法,代码如下:
package com.example.listview.adapter
import android.content.Context
import android.support.annotation.LayoutRes
import android.support.annotation.NonNull
import android.support.annotation.Nullable
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.ArrayAdapter
import android.widget.ImageView
import android.widget.TextView
import com.example.listview.R
import com.example.listview.vo.Things
import java.util.List
public class ThingsAdapter extends ArrayAdapter {
private int resourceId
public ThingsAdapter(@NonNull Context context, @LayoutRes int textViewResourceId, @NonNull List objects) {
super(context, textViewResourceId, objects)
resourceId = textViewResourceId
}
@NonNull
@Override
public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) {
Things things = getItem(position)
View view = LayoutInflater.from(getContext()).inflate(resourceId,parent,false)
ImageView thingsImg = (ImageView) view.findViewById(R.id.thing_img)
TextView thingName = (TextView) view.findViewById(R.id.thing_name)
thingsImg.setImageResource(things.getImgId())
thingName.setText(things.getName())
return view
}
}
- 第五步、MainActivity中调用自定义适配器,并且向ListView中逐条新增数据,代码如下:
package com.example.listview
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.widget.ListView
import com.example.listview.adapter.ThingsAdapter
import com.example.listview.vo.Things
import java.util.ArrayList
import java.util.List
public class MainActivity extends AppCompatActivity {
private List thingsList = new ArrayList<>()
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
initThings()
ThingsAdapter adapter = new ThingsAdapter(MainActivity.this,R.layout.things_item,thingsList)
ListView listView = (ListView) findViewById(R.id.list_view)
listView.setAdapter(adapter)
}
private void initThings() {
for (int i = 0
Things phOne= new Things("Phone",R.mipmap.ic_launcher)
thingsList.add(phone)
Things tv = new Things("Tv",R.mipmap.ic_launcher)
thingsList.add(tv)
Things cell = new Things("cell",R.mipmap.ic_launcher)
thingsList.add(cell)
Things apple = new Things("Apple",R.mipmap.ic_launcher)
thingsList.add(apple)
}
}
}
以上就实现我们的适配器,Looper虽长,但总还算是圆的,感谢大家的支持!!!