//NGUI............................................................
using System;
using UnityEngine;
using System.Collections;
public class ScrollViewTurnPage : MonoBehaviour
{
[Header("一页的宽度,一般就是ScrollView的宽度")]
[SerializeField] private int m_pageWidth = 600;
[Range(5.0f, 55.0f)]
[SerializeField] private int m_springStrength = 35;
private int m_totalPages = 4; //固定的时候,写的页数
[Header("列数")]
[SerializeField] private int m_columns = 4;
[Header("行数")]
[SerializeField] private int m_row = 3;
#region Public Interface
public void PreviousPage()
{
if (m_bHorizontal)
{
if (m_currPage > 1) Page(m_pageWidth);
}
else
{
if (m_currPage }
}
public void NextPage()
{
if (m_bHorizontal)
{
if (m_currPage }
else
{
if (m_currPage > 1) Page(-m_pageWidth);
}
}
public int CurrentPage
{
get { return m_currPage; }
}
public int TotalPages
{
get { return m_totalPages; }
set { m_totalPages = value; }
}
public int OnePageItemsMaxNum
{
get { return m_columns * m_row; }
}
public int ItemColumns
{
get { return m_columns; }
}
public int PageWidth
{
get { return m_pageWidth; }
}
#endregion
#region private interface
private int m_currPage = 1;
private UIScrollView m_scrollView = null;
private float m_nowLocation = 0;
private bool m_bDrag = false;
private bool m_bSpringMove = false;
private SpringPanel m_springPanel = null;
private bool m_bHorizontal = true;
void Awake()
{
m_scrollView = gameObject.GetComponent();
if (m_scrollView == null)
m_scrollView = gameObject.AddComponent();
m_springPanel = GetComponent();
if (m_springPanel == null) m_springPanel = gameObject.AddComponent();
m_springPanel.enabled = true;
m_scrollView.onDragStarted = OnDragStarted;
m_scrollView.onMomentumMove = onMomentumMove;
m_scrollView.onStoppedMoving = onStoppedMoving;
m_scrollView.onDragFinished = onDragFinished;
m_bHorizontal = m_scrollView.movement == UIScrollView.Movement.Horizontal ? true : false;
onStoppedMoving();
}
void onDragFinished()
{
onMomentumMove();
}
void OnDragStarted()
{
m_bDrag = false;
SetNowLocation();
}
void onMomentumMove()
{
if (m_bDrag) return;
Vector3 v3 = transform.localPosition;
float value = 0;
if (m_bHorizontal)
{
value = m_nowLocation - v3.x;
//if (Mathf.Abs(value) if (value > 0)
{
if (m_currPage }
else
{
if (m_currPage > 1) Page(m_pageWidth);
}
}
else
{
value = m_nowLocation - v3.y;
//if (Mathf.Abs(value) if (value > 0)
{
if (m_currPage > 1) Page(-m_pageWidth);
}
else
{
if (m_currPage }
}
}
void Page(float value)
{
m_bSpringMove = true;
m_bDrag = true;
//m_springPanel = GetComponent();
//if (m_springPanel == null) m_springPanel = gameObject.AddComponent();
m_springPanel.enabled = false;
Vector3 pos = m_springPanel.target;
pos = m_bHorizontal ? new Vector3(pos.x + value, pos.y, pos.z) : new Vector3(pos.x, pos.y + value, pos.z);
if (!SetIndexPage(pos)) return;
SpringPanel.Begin(gameObject, pos, m_springStrength).strength = 10.0f;
m_springPanel.onFinished = SpringPanleMoveEnd;
//Debug.Log("current Page ==" + m_currPage);
}
void SpringPanleMoveEnd()
{
m_bSpringMove = false;
//重新定位
}
void onStoppedMoving()
{
m_bDrag = false;
SetNowLocation();
}
void SetNowLocation()
{
if (m_bHorizontal)
{
m_nowLocation = gameObject.transform.localPosition.x;
}
else
{
m_nowLocation = gameObject.transform.localPosition.y;
}
}
bool SetIndexPage(Vector3 v3)
{
float value = m_bHorizontal ? v3.x : v3.y;
//if (m_bHorizontal)
//{
// if (value > 0 || value <(m_totalPages) * -m_pageWidth) return false;
//}
//else
//{
// if (value <0 || value > (m_totalPages - 1) * m_pageWidth) return false;
//}
value &#61; Mathf.Abs(value);
m_currPage &#61; (int) (value / m_pageWidth) &#43; 1;
return true;
}
#endregion
}
简单的实现了下NGUI的上下翻页。有两个接口&#xff0c;分别是上一页和下一页。
用法如下。

如上图&#xff0c;第一个参数为 Page的宽或高&#xff0c;具体由你的滑动组件设定的方向定。
第二个参数为滑动系数&#xff0c;即&#xff0c;滑动了多远就翻页
第三个参数为总页数。
依赖组件。UIScrollView&#xff0c;UICenterOnChild。
脚本和Scroll一起
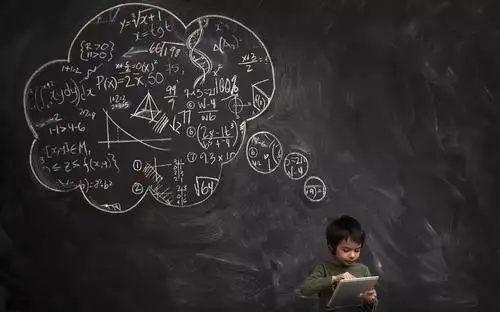
分页父物体绑上居中脚本。为Scroll的子物体
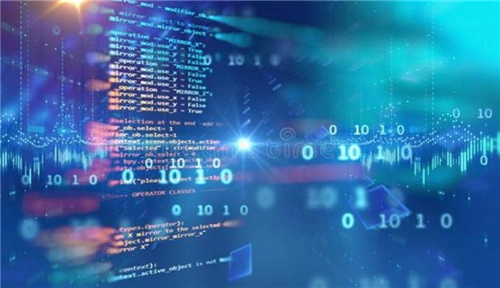
这个基本功能没问题。但是有一个小缺陷。就是Panel的坐标必须为中心点。如果需要位移需要再加一个父物体。让滑动组件依赖的Panel的相对坐标都为0.
原因是因为我用的坐标去计算的当前页。大家如果有空。可以改为偏移量就能解决这问题。
因为我也用不上这个。这个是写给学员参考的。所以就懒得改了。大家需要的改的话。可以自行修改。
最后。上脚本。
- usingUnityEngine;
- usingSystem.Collections;
-
- publicclassYouKeTurnPage:MonoBehaviour
- {
- ///
- /// 每页宽度(游-客-学-院)
- ///
- publicfloat pageWidth;
- ///
- /// 翻页力度(游.客.学.院)
- ///
- publicintEffortsFlip&#61;50;
- ///
- /// 总页数
- ///
- publicint pageNums&#61;0;
-
- ///
- /// 当前所在页
- ///
- publicint pageIndex
- {
- get
- {
- return mPageIndex;
- }
- }
- ///
- /// 当前所在页
- ///
- privateint mPageIndex&#61;1;
- privateUIScrollView mScrollView&#61;null;
- privatefloat nowLocation&#61;0;
- private bool isDrag&#61;false;
- private bool isSpringMove&#61;false;
- privateSpringPanel mSp&#61;null;
- private bool isHorizontal&#61;true;
-
- voidAwake()
- {
- mScrollView&#61; gameObject.GetComponent();
- if(mScrollView&#61;&#61;null)
- {
- mScrollView&#61; gameObject.AddComponent();
- }
-
- mScrollView.onDragStarted&#61;OnDragStarted;
- mScrollView.onMomentumMove&#61; onMomentumMove;
- mScrollView.onStoppedMoving&#61; onStoppedMoving;
- if(mScrollView.movement&#61;&#61;UIScrollView.Movement.Horizontal)
- {
- isHorizontal&#61;true;
- }
- else
- {
- isHorizontal&#61;false;
- }
- onStoppedMoving();
- }
-
- voidOnDragStarted()
- {
- isDrag&#61;false;
- SetNowLocation();
- }
-
- void onMomentumMove()
- {
- if(isDrag)return;
- Vector3 v3&#61; transform.localPosition;
- float value&#61;0;
- if(isHorizontal)
- {
- value&#61; nowLocation- v3.x;
- if(Mathf.Abs(value)<EffortsFlip)return;
- if(value>0)
- {
- if(mPageIndex< pageNums)Page(-pageWidth);
- }
- else
- {
- if(mPageIndex>1)Page(pageWidth);
- }
- }
- else
- {
- value&#61; nowLocation- v3.y;
- if(Mathf.Abs(value)<EffortsFlip)return;
- if(value>0)
- {
- if(mPageIndex>1)Page(-pageWidth);
- }
- else
- {
- if(mPageIndex< pageNums)Page(pageWidth);
- }
- }
- }
-
- voidPage(float value)
- {
- isSpringMove&#61;true;
- isDrag&#61;true;
- mSp&#61;GetComponent();
- if(mSp&#61;&#61;null)mSp&#61; gameObject.AddComponent();
- //mSp.enabled &#61; false;
- Vector3 pos&#61; mSp.target;
- pos&#61; isHorizontal?newVector3(pos.x&#43; value, pos.y, pos.z):newVector3(pos.x, pos.y&#43; value, pos.z);
- if(!SetIndexPage(pos))return;
- SpringPanel.Begin(gameObject, pos,13f).strength&#61;8f;
- mSp.onFinished&#61;SpringPanleMoveEnd;
- Debug.Log("page index&#61;"&#43;mPageIndex);
- }
-
- voidSpringPanleMoveEnd()
- {
- isSpringMove&#61;false;
- }
-
- void onStoppedMoving()
- {
- isDrag&#61;false;
- SetNowLocation();
- }
-
- voidSetNowLocation()
- {
- if(isHorizontal)
- {
- nowLocation&#61; gameObject.transform.localPosition.x;
- }
- else
- {
- nowLocation&#61; gameObject.transform.localPosition.y;
- }
- }
-
- boolSetIndexPage(Vector3 v3)
- {
- float value&#61; isHorizontal? v3.x: v3.y;
- //Debug.Log((pageNums - 1) * pageWidth);
- if(isHorizontal)
- {
- if(value>0|| value<(pageNums-1)*-pageWidth)returnfalse;
- }
- else
- {
- if(value<0|| value>(pageNums-1)* pageWidth)returnfalse;
- }
-
- value&#61;Mathf.Abs(value);
- mPageIndex&#61;(int)(value/ pageWidth)&#43;1;
-
- returntrue;
- }
-
- #region 公共接口 游*客*学*院
-
- ///
- /// 上一页
- ///
- publicvoidPreviousPage()
- {
- if(isHorizontal)
- {
- if(mPageIndex>1)Page(pageWidth);
- }
- else
- {
- if(mPageIndex< pageNums)Page(pageWidth);
- }
- }
-
- ///
- /// 下一页
- ///
- publicvoidNextPage()
- {
- if(isHorizontal)
- {
- if(mPageIndex< pageNums)Page(-pageWidth);
- }
- else
- {
- if(mPageIndex>1)Page(-pageWidth);
- }
- }
- #endregion
- }
//UGUI..............................................\
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
using UnityEngine.UI;
public class GridItem {
public string cnName;
public string usName;
public GridItem(string cnN,string usN) {
cnName &#61; cnN;
usName &#61; usN;
}
}
public class PaginationPanel : MonoBehaviour
{
///
/// 当前页面索引
///
public int m_PageIndex &#61; 1;
///
/// 总页数
///
public int m_PageCount &#61; 0;
///
/// 每页元素数
///
public int m_PerPageCount &#61; 0;
///
/// 元素总个数
///
public int m_ItemsCount &#61; 0;
///
/// 元素列表
///
public List m_ItemsList;
///
/// 上一页
///
public Button m_BtnPrevious;
///
/// 下一页
///
public Button m_BtnNext;
///
/// 显示当前页数的标签
///
public Text m_PanelText;
public Transform m_FrontPanel;
public Transform m_BackPanel;
public bool m_IsTouching;
[Range(-1,1)]
public float m_TouchDelta;
public float m_MoveSpeed &#61; 5f;
public float m_FadeDistance;
public Vector3 m_PrePos;
public Vector3 m_CenterPos;
public Vector3 m_NextPos;
public bool m_DoPrevious;
public bool m_DoNext;
public float m_CurrAlpha;
private bool m_HasPrevious;
private bool m_HasNext;
private bool m_IsSaveBeforeTouch;
public int m_FrontPanelPageIndex;
private bool m_CanChangePage;
private bool m_IsBackPageIndex;
void Start()
{
InitGUI();
InitItems();
}
///
/// 初始化GUI
///
private void InitGUI()
{
//m_BtnNext &#61; GameObject.Find("Canvas/Panel/BtnNext").GetComponent